This function will write out all sketches in the currently active plane to a script file that can later be read back in using "Run Script". It outputs all points, edges, dimensions, and constraints for sketches in the plane. However it does not output any plane boundary edges, or dimensions or constraints that reference boundary edges or their endpoints. Sketch Instances are output as normal sketches.
The output file contains a function definition where all of the sketch items are created and then a call to that function. This format was chosen to make it easier for you to cut/paste to combine several files, while only making minor changes. Also, all edges and dimensions can be accessed via the returned value from the function call. Also, the "with (p.Sk1)" and "with (p.plane)" blocks are used to avoid having to include that part of the identifier in function calls. For example:
with (p.Sk1)
{
p.Ln5 = Line(3, 4, 5, 6);
p.Ln6 = Line(9, 8, 7, 6)
}
Is the same as:
p.Ln5 = p.Sk1.Line(3, 4, 5, 6);
p.Ln6 = p.Sk1.Line(9, 8, 7, 6);
Also note that geometry is written out in only one format. How the geometry was created does not effect the output. For example an Arc can be created via a number of methods, including Fillet or even splitting or trimming a full circle. However, no matter how the Arc is created, it is output via the ArcCtrEdge command.
Example of a fully dimensioned sketch:
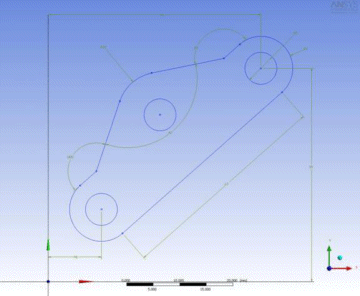
Below is an example script output for the above sketch.
Note: The Write Script function, when performed in the current release of the Ansys DesignModeler application, will reflect the appropriate release number.
//DesignModeler JScript, version: Ansys DesignModeler 11.0 (Sep 6 2005, 09:59:59; 11,2005,247,1, DEBUG) SV4 //Created via: "Write Script: Sketch(es) of Active Plane" // Written to: E:\SketchExample2.js // On: 09/06/05, 10:14:16 //Using: // agb ... pointer to batch interface //Note: // You may be able to re-use below JScript function via cut-and-paste; // however, you may have to re-name the function identifier. // function planeSketchesOnly (p) { //Plane p.Plane = agb.GetActivePlane(); p.Origin = p.Plane.GetOrigin(); p.XAxis = p.Plane.GetXAxis(); p.YAxis = p.Plane.GetYAxis(); //Sketch p.Sk1 = p.Plane.newSketch(); p.Sk1.Name = "Sketch1"; //Edges with (p.Sk1) { p.Pt16 = ConstructionPoint(21.0316, 31.2714); p.Pt17 = ConstructionPoint(9.03138, 20.6882); p.Pt18 = ConstructionPoint(33.0314, 41.8542); with (p.Plane) { p.Pt16 = ConstructionPoint(21.0316, 31.2714); } with (p.Plane) { p.Pt17 = ConstructionPoint(9.03138, 20.6882); } with (p.Plane) { p.Pt18 = ConstructionPoint(33.0314, 41.8542); } p.Ln7 = Line(13.9686, 9.0425, 43.9686, 35.5); p.Cr8 = ArcCtrEdge( 40, 40, 43.9686, 35.5, 36.0314, 44.5); p.Ln9 = Line(36.0314, 44.5, 33.0314, 41.8542); p.Cr10 = ArcCtrEdge( 10, 13.5425, 6.03139, 18.0425, 13.9686, 9.0425); p.Cr11 = Circle(40, 40, 3); p.Cr12 = Circle(10, 13.5425, 3); p.Ln13 = Line(9.03138, 20.6882, 6.03139, 18.0425); p.Ln14 = Line(9.03138, 20.6882, 13.449, 33.8219); p.Ln15 = Line(33.0314, 41.8542, 19.4491, 39.1133); p.Cr16 = ArcCtrEdge( 21.0316, 31.2714, 19.4491, 39.1133, 13.449, 33.8219); p.Cr16.DeleteCenter(); p.Cr17 = Circle(21.0316, 31.2714, 3); } //Dimensions and/or constraints with (p.Plane) { //Dimensions var dim; dim = HorizontalDim(p.Cr8.Center, 40, 40, p.Origin, 0, 0, 21.428, 50.0367); if(dim) dim.Name = "H4"; dim = HorizontalDim(p.Cr10.Center, 10, 13.5425, p.Origin, 0, 0, 5.27234, 4.61012); if(dim) dim.Name = "H6"; dim = VerticalDim(p.Cr8.Center, 40, 40, p.Origin, 0, 0, 49.5663, 21.5408); if(dim) dim.Name = "V5"; dim = DistanceDim(p.Ln7.End, 43.9686, 35.5, p.Ln7.Base, 13.9686, 9.0425, 33.701, 18.3564); if(dim) dim.Name = "L3"; dim = RadiusDim(p.Cr8, 48.6124, 43.7176, 0); if(dim) dim.Name = "R1"; dim = RadiusDim(p.Cr16, 10.5152, 43.9831, 0); if(dim) dim.Name = "R10"; dim = DiameterDim(p.Cr11, 46.5855, 46.6387, 0); if(dim) dim.Name = "D2"; dim = AngleDim(p.Ln14, 9.03138, 20.6882, p.Ln15, 33.0314, 41.8542, 23.065, 27.9382); if(dim) dim.Name = "A7"; dim = AngleDim(p.Ln9, 36.0314, 44.5, p.Ln15, 19.4491, 39.1133, 28.8903, 45.2907); if(dim) dim.Name = "A8"; dim = AngleDim(p.Ln14, 13.449, 33.8219, p.Ln13, 6.03139, 18.0425, 4.43777, 23.3889); if(dim) { dim.DimRefFlag = agc.Yes; dim.Name = "A9"; } //Constraints TangentCon(p.Cr8, 43.9686, 35.5, p.Ln7, 44.4721, 35.5279); TangentCon(p.Cr8, 36.0314, 44.5, p.Ln9, 35.5279, 44.4721); TangentCon(p.Cr10, 6.03139, 18.0425, p.Ln13, 5.52786, 14.4721); TangentCon(p.Cr10, 13.9686, 9.0425, p.Ln7, 14.4721, 5.52786); TangentCon(p.Cr16, 19.4491, 39.1133, p.Ln15, 17.8038, 37.9547); TangentCon(p.Cr16, 13.449, 33.8219, p.Ln14, 14.8038, 35.309); CoincidentCon(p.Ln7.End, 43.9686, 35.5, p.Cr8.Base, 43.9686, 35.5); CoincidentCon(p.Cr8.End, 36.0314, 44.5, p.Ln9.Base, 36.0314, 44.5); CoincidentCon(p.Ln13.End, 6.03139, 18.0425, p.Cr10.Base, 6.03139, 18.0425); CoincidentCon(p.Cr10.End, 13.9686, 9.0425, p.Ln7.Base, 13.9686, 9.0425); CoincidentCon(p.Cr11.Center, 40, 40, p.Cr8.Center, 40, 40); CoincidentCon(p.Cr12.Center, 10, 13.5425, p.Cr10.Center, 10, 13.5425); CoincidentCon(p.Ln9, 21.0314, 31.2712, p.Ln13, 21.0314, 31.2712); CoincidentCon(p.Pt17, 9.03138, 20.6882, p.Ln13, 15.0238, 25.973); CoincidentCon(p.Pt18, 33.0314, 41.8542, p.Ln9, 27.2306, 36.7385); CoincidentCon(p.Ln14.Base, 9.03138, 20.6882, p.Pt17, 9.03138, 20.6882); CoincidentCon(p.Ln15.Base, 33.0314, 41.8542, p.Pt18, 33.0314, 41.8542); CoincidentCon(p.Pt17, 9.03138, 20.6882, p.Ln13.Base, 9.03138, 20.6882); CoincidentCon(p.Pt16, 21.0316, 31.2714, p.Ln9, 21.0314, 31.2712); CoincidentCon(p.Pt18, 33.0314, 41.8542, p.Ln9.End, 33.0314, 41.8542); CoincidentCon(p.Ln15.End, 19.4491, 39.1133, p.Cr16.Base, 19.4491, 39.1133); CoincidentCon(p.Ln14.End, 13.449, 33.8219, p.Cr16.End, 13.449, 33.8219); CoincidentCon(p.Cr17.Center, 21.0316, 31.2714, p.Pt16, 21.0316, 31.2714); CoincidentCon(p.Cr17.Center, 21.0316, 31.2714, p.Ln13, 8.75223, 20.4421); ParallelCon(p.Ln7, p.Ln13); ConcentricCon(p.Cr17, p.Cr16); EqualRadiusCon(p.Cr8, p.Cr10); EqualRadiusCon(p.Cr12, p.Cr11); EqualRadiusCon(p.Cr17, p.Cr11); EqualLengthCon(p.Ln13, p.Ln9); } p.Plane.EvalDimCons(); //Final evaluate of all dimensions and constraints in plane return p; } //End Plane JScript function: planeSketchesOnly //Call Plane JScript function var ps1 = planeSketchesOnly (new Object()); //Finish agb.Regen(); //To insure model validity //End DM JScript
Other file management options: