
This section describes how you can add a button to your interface.
While dialog boxes come with standard cx-create-button
macro.
(cx-create-button parent label callback row column)
Argument | Type | Description |
---|---|---|
parent | object | The name of the table that you are adding the button to |
label | string | The name of the button to be displayed on the GUI |
callback | symbol/function | The name of the function called when the button is clicked |
row | symbol/int | When added to a table, signifies the row that the button is added to |
column | symbol/int | When added to a table, signifies the column that the button is added to |
Note: The row and column attributes are optional. If you leave out one or both of these attributes the button will be added to the first row/column of the parent attribute and overwrite anything that is already in that spot.
This example shows how the cx-create-button
macro is used to create a new button, and how the callback argument
works. In the cx-create-button
statement
below, the 'activate-callback button-cb
argument
ensures that the button-cb
procedure is called
each time that the button is clicked. The button-cb
function is set up with the line (define (button-cb
. args)
, which is just like how the apply-cb
and update-cb
functions are set up in a
fully functional dialog box (see Comprehensive Examples). Once you open the button-cb
procedure with this line you can then write
the code to give it functionality.
In this example, the variable counter
is incremented by 1
each time the button
is clicked and the number of times that the button has been clicked
is output to a text entry field txtField
.
This dialog box does not do anything when the button is clicked because we have substituted Boolean values for
the apply-cb
and update-cb
arguments, which would normally be function calls like the button-cb
argument is in the cx-create-button
line. For more information on the apply-cb
and update-cb
functions, see cx-create-panel.
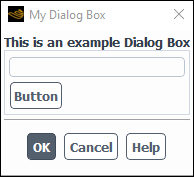
(define (apply-cb) #t) (define update-cb #f) (define table) (define txtField) (define counter 0) (define (button-cb . args) (set! counter (+ counter 1)) (cx-set-text-entry txtField (string-append "Times Clicked: " (number->string counter))) ) (define my-dialog-box (cx-create-panel "My Dialog Box" apply-cb update-cb)) (set! table (cx-create-table my-dialog-box "This is an example Dialog Box")) (set! txtField (cx-create-text-entry table "" 'row 0 'col 0)) (cx-create-button table "Button" 'activate-callback button-cb 'row 1 'col 0) (cx-show-panel my-dialog-box)
To view additional examples of buttons, see Comprehensive Examples.