This section describes how you can create an interface in Fluent using a dialog box.
The process that you will create a user interface to correspond
with a user-defined function is to create a dialog box. Dialog boxes
are created using the cx-create-panel
macro.
Once a dialog box is created, you must use the cx-show-panel
macro to display it. Once you are able to create a simple dialog
box, it will be easier to learn how to add more complex elements to
it. Example One illustrates the simplest
way to create a dialog box. It should be noted that this dialog box
does not have any functionality. Example Two adds additional GUI elements but still lacks functionality. Dialog
boxes use tables to format interface elements. For more information
on tables, see Tables (cx-create-table). For more on the
individual GUI elements seen in these examples, see Interface Elements.
This section explains the arguments used in the various dialog box macros.
(cx-create-panel title apply-cb update-cb)
Argument | Type | Description |
---|---|---|
title | string | Name of the dialog box |
apply-cb | function | Name of the function called when the | button is clicked
update-cb | function | Name of the function called when the dialog box is opened |
Note: While the apply-cb
and update-cb
arguments are normally function names that
are called when the dialog box is opened or the button is clicked, they do not need to be function names for the cx-create-panel
macro to work. In many examples throughout
part two of this guide, the apply-cb
and update-cb
arguments will be Boolean values rather than
function names. The examples that use Boolean values instead of function
names do not have any use when the button
is clicked, but they do allow us to focus more on the specific elements
of the interface rather than the overhead of setting up these functions.
For examples where the apply-cb
and update-cb
arguments are set up as functions and allow
functionality through RP variables, see Comprehensive Examples.
This section provides examples of dialog boxes being created in Fluent.
This is an example of a very simple dialog box containing only
an empty table. The apply-cb
and update-cb
arguments must be included in the cx-create-panel
statement, but in this case they have
been simplified to just Boolean values rather than functions. Note
that the apply-cb
variable must be #t
if you use a Boolean value instead of a function,
because using #f
will remove the button from the dialog box. Due to the lack of any
data input fields on the dialog box and the use of Boolean values
in the place of function calls, this dialog box does not serve any
purpose, and is intended to simply illustrate the process of creating
a dialog box.
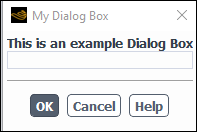
(define (apply-cb) #t) (define update-cb #f) (define my-dialog-box (cx-create-panel "My Dialog Box" apply-cb update-cb)) (define table) (set! table(cx-create-table my-dialog-box "This is an example Dialog Box")) (cx-show-panel my-dialog-box)
This is an example of a dialog box with a few basic data input
fields added. The apply-cb
and update-cb
arguments must be included in the cx-create-panel
statement, but in this case they have
been simplified to just Boolean values rather than functions. Note
that the apply-cb
variable must be #t
if you use a Boolean value instead of a function,
because using #f
will remove the button from the dialog box. Due to the use of boolean
values in the place of function calls, this dialog box does not serve
any purpose, and is intended to simply illustrate the process of creating
a dialog box with various data input fields.
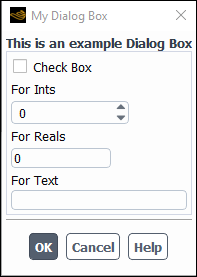
(define (apply-cb) #t) (define update-cb #f) (define table) (define checkbox) (define ints) (define reals) (define txt) (define my-dialog-box (cx-create-panel "My Dialog Box" apply-cb update-cb)) (set! table (cx-create-table my-dialog-box "This is an example Dialog Box")) (set! checkbox (cx-create-toggle-button table "Check Box" 'row 0)) (set! ints (cx-create-integer-entry table "For Ints" 'row 1)) (set! reals (cx-create-real-entry table "For Reals" 'row 2)) (set! txt (cx-create-text-entry table "For Text" 'row 3)) (cx-show-panel my-dialog-box)
For further examples of dialog boxes with additional elements, see Comprehensive Examples.