This section describes how you can create an interface in Fluent using a task page.
Another method of displaying GUI elements in Fluent is by using a task page. Task pages
are different from dialog boxes because a task page is integrated directly into the Fluent
interface rather than displaying your interface via a new window. This is helpful if you are
working with several applications at once and don’t have the screen space for a whole new
UDF window. Task pages are very similar to dialog boxes, and are created using the
cx-create-taskpage
macro. Once a task page is created, you must use
the cx-show-taskpage
macro to display it. Example One illustrates the simplest way to create a task page. It should
be noted that this task page does not have any functionality. Example Two adds additional GUI elements but still lacks functionality.
Task pages use tables to format interface elements. For more information on tables, see Tables (cx-create-table).
Note: The current task page selected in the selection screen on the left-most part of the Fluent interface does not affect your task page being opened.
This section explains the arguments used in the various task page macros.
(cx-create-taskpage title apply-cb update-cb)
Argument | Type | Description |
---|---|---|
title | string | Name of the task page |
apply-cb | function | Name of the function called when the | button is clicked
update-cb | function | Name of the function called when the task page is opened |
Note: While the apply-cb
and update-cb
arguments are normally function names that are called when the task page is opened or the
button is clicked, they do not need to be function names for the
cx-create-taskpage
macro to work. In many examples throughout part
two of this guide, the apply-cb
and
update-cb
arguments will be Boolean values rather than function
names. The examples that use Boolean values instead of function names do not have any use when
the button is clicked, but they do allow us to focus more on the
specific elements of the interface rather than the overhead of setting up these functions. For
examples where the apply-cb
and update-cb
arguments are set up as functions and allow functionality through RP variables, see Comprehensive Examples.
This section provides examples of task pages being created in Fluent.
This is an example of a very simple task page containing only an empty table. The
apply-cb
and update-cb
arguments must be
included in the cx-create-taskpage
statement, but in this case they
have been simplified to just Boolean values rather than functions. Note that the
apply-cb
variable must be #t
if you use a
Boolean value instead of a function, because using #f
will remove the
button from the task page. Due to the lack of any data input
fields on the dialog box and the use of Boolean values in the place of function calls, this
task page does not serve any purpose, and is intended to simply illustrate the process of
creating a task page.
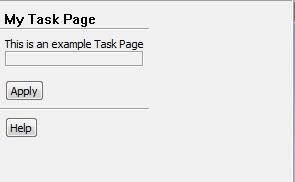
(define (apply-cb) #t) (define update-cb #f) (define my-taskpage (cx-create-taskpage "My Task Page" apply-cb update-cb)) (define table) (set! table (cx-create-table my-taskpage "This is an example Task Page")) (cx-show-taskpage my-taskpage)
This is an example of a task page with a few basic data input fields added. The
apply-cb
and update-cb
arguments must be
included in the cx-create-taskpage
statement, but in this case they
have been simplified to just Boolean values rather than functions. Note that the
apply-cb
variable must be #t
if you use a
Boolean value instead of a function, because using #f
will remove the
button from the task page. Due to the use of Boolean values in
the place of function calls, this task page does not serve any purpose, and is intended to
simply illustrate the process of creating a task page with various data input fields.
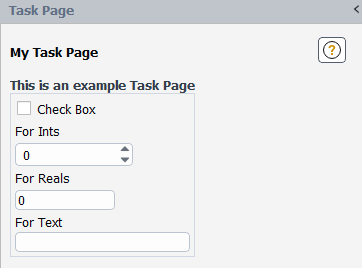
(define (apply-cb) #t) (define update-cb #f) (define table) (define checkbox) (define ints) (define reals) (define txt) (define my-task-page (cx-create-taskpage "My Task Page" apply-cb update-cb)) (set! table (cx-create-table my-task-page "This is an example Task Page")) (set! checkbox (cx-create-toggle-button table "Check Box" 'row 0)) (set! ints (cx-create-integer-entry table "For Ints" 'row 1)) (set! reals (cx-create-real-entry table "For Reals" 'row 2)) (set! txt (cx-create-text-entry table "For Text" 'row 3)) (cx-show-taskpage my-task-page)
For further examples of task pages with additional elements, see Comprehensive Examples.