The following routines define the topology of elements (using node IDs) that are to be imported to CFX. Also included here are routines that get the local face number and vertices of an element.
ID_t cfxImportElement (elemid, elemtype, nodelist) ID_t elemid, *nodelist; int elemtype;
Define a new element to be imported to CFX. The unique identifier
of the element is given by elemid
, the element
type by elemtype
and the list of vertices by nodelist
. If an element with the same ID has already been
defined, it will be replaced by the new element being defined.
Only volume elements are currently supported by CFX; these may
be tetrahedrons (4 vertices), pyramids (5 vertices), prisms (6 vertices)
or hexahedrons (8 vertices). elemtype
is the
number of vertices for the element.
The following defines are included in the header file, cfxImport.h for convenience:
#define cfxELEM_TET 4 /* tet element (4 nodes) */ #define cfxELEM_PYR 5 /* pyramid element (5 nodes) */ #define cfxELEM_WDG 6 /* wedge element (6 nodes) */ #define cfxELEM_HEX 8 /* hex element (8 nodes) */
The list of vertices in nodelist
refers
to IDs of nodes that on termination of the import program by a call
to cfxImportDone
must have been defined by calls
to cfxImportNode
. If this is not the case a fatal
error will be reported and the API will terminate.
The vertex ordering for the elements follows Patran Neutral File element conventions, and is shown in the following figure.
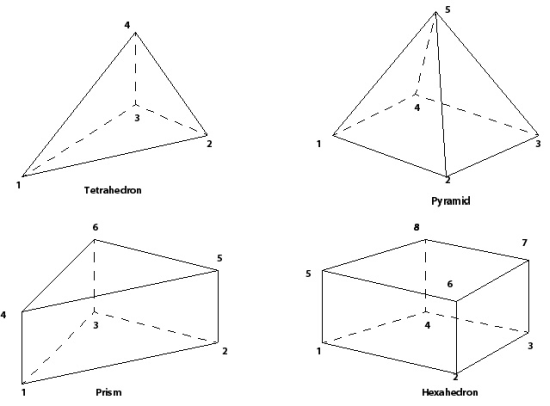
Returns 0 in the case of an elemid
is invalid
(less than 1) or an unsupported value is given by elemtype
, or elemid
if the element is successfully defined.
If the element already exists the vertices of the element will be
redefined.
ID_t cfxImportGetElement (elemid, nodelist) ID_t elemid, nodelist[];
Get the node IDs for corresponding to the vertices of element
identified by elemid
and store in the array nodelist.
This array must be at least as large the number of vertices for the
element (a size of 8 will handle all possible element types).
Returns 0 if the element is not defined, or the element type
(number of vertices). The node IDs will be ordered in the order expected
by cfxImportElement
if the program was to redefine
the element.
ID_t * cfxImportElementList ()
Returns an array of all the currently defined element IDs or NULL if no elements have been defined. The first entry in the array is the number of elements.
The memory for the array returned is allocated using malloc
by the routine, consequently it should be destroyed
when no longer required by calling free
.
ID_t cfxImportGetFace (elemid, facenum, nodelist) ID_t elemid, nodelist[]; int facenum;
Gets the node IDs for the local facenum
’th face of the element identified by elemid
.
The node IDs are returned in nodelist
,
which should be of at least of size 4. The nodes correspond to the
vertices of the face and are ordered counter-clockwise such that the
normal for the face points away from the element. The face numbers
and associated node indices are modeled after Patran Neutral File
elements, and are tabulated here:
Element Type | Face | Nodes | |||
---|---|---|---|---|---|
tetrahedron | 1 | 1 | 3 | 2 |
|
| 2 | 1 | 2 | 4 |
|
| 3 | 2 | 3 | 4 |
|
| 4 | 1 | 4 | 3 |
|
pyramid | 1 | 1 | 4 | 3 | 2 |
| 2 | 1 | 2 | 5 |
|
| 3 | 2 | 3 | 5 |
|
| 4 | 3 | 4 | 5 |
|
| 5 | 1 | 5 | 4 |
|
prism | 1 | 1 | 3 | 2 |
|
| 2 | 4 | 5 | 6 |
|
| 3 | 1 | 2 | 5 | 4 |
| 4 | 1 | 4 | 6 | 3 |
| 5 | 2 | 3 | 6 | 5 |
hexahedron | 1 | 1 | 2 | 6 | 5 |
| 2 | 3 | 4 | 8 | 7 |
| 3 | 1 | 4 | 3 | 2 |
| 4 | 2 | 3 | 7 | 6 |
| 5 | 5 | 6 | 7 | 8 |
| 6 | 1 | 5 | 8 | 4 |
Note: The face numbers and associated node indices are different when exporting elements. For details, see cfxExportFaceNodes.
Returns -1 if the element has not been defined, 0 if the face number is out of range, or the number of nodes for the face (3 or 4):
ID_t cfxImportFindFace (elemid, nnodes, nodeid) ID_t elemid, nodeid[]; int nnodes;
Gets the local face number in element identified by elemid
that contains all the nodes supplied by the calling
routine in nodeid
. nnodes
is the number of nodes for the face (3 or 4).
Returns -1 if the element is not found or nodeid
is not supplied or nnodes
is greater than 4
or less than 3. Returns 0 if there is no match, or the local face
number (1 to 6) of the element.