Many of Fluent Aero's features and settings can be accessed and changed from the console using Python commands.
Many of the commands and settings done in Fluent Aero panels can be recorded to python commands, by using the
→ menu, and can also be executed through the → menu.The same commands can then be used to automate Fluent Aero, by launching it in batch mode, as described in Batch Launching.
Python Console & Operations
The Console window at the bottom right of Fluent Aero permits you to enter commands interactively. The following sections describe example commands available in the Python console.
dir() and the command/attribute list
dir()
Display the list of global commands and top-level objects.
'AddSession', 'Project', 'ReadScriptFile', 'Sim', 'StartTranscript', 'StopTranscript', 'csim'
dir(object)
Display the list of commands & attributes of the object.
Example 3.2: Entering Commands Interactively (Assuming airfoil-demo is already loaded) dir(Sim[“airfoil-demo”])
dir(Sim[“airfoil-demo”])
'AeroWorkflow', 'Connect', 'ConnectionInfo', 'Disconnect', ‘SendCommand’
r= csim()
A temporary variable can be used to simplify command lines.
dir(r.AeroWorkflow.Setup.GeometricProperties)
'DomainType', 'DragDir', 'LiftDir', ‘RefArea', 'RefLength', `RefreshBCs’
Accessing/Changing values
The () operator will return the current value of the attribute.
Result: 1
The= operator will set the current value of the attribute.
r.AeroWorkflow.Setup.GeometricProperties.RefArea= 0.05
Commands
If an item listed in dir() is a verb, it is a command. For example:
r.AeroWorkflow.Setup.GeometricProperties.RefreshBCs()
Sample Script
Note: The following two lines are equivalent when connected to a single
simulation, therefore, csim()
can be used as a
shorthand operation:
r = RemoteSession[“RemoteSession1”]
r = csim()
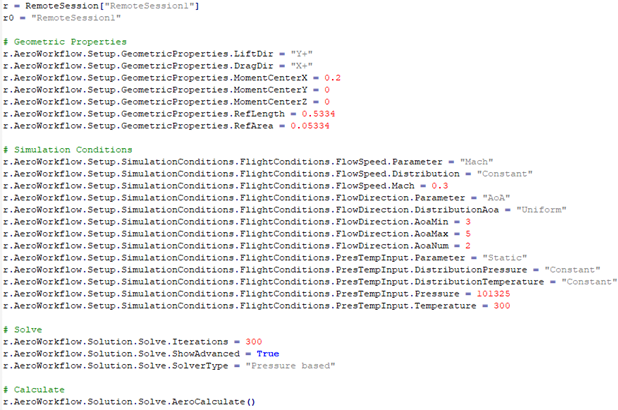
Fluent Aero’s object data structure (accessible from the python console) is
organized similarly to the object nodes shown in the Outline
View. The first level is the simulation session id (example:
“RemoteSession1”
, or
r=RemoteSession[“RemoteSession1”]
, or
r=csim()]
, followed by the Fluent Aero workflow container
object [example: r.AeroWorkflow()
]. After that, most of the
objects and commands are organized exactly like shown in the Outline
View, following the structure:
RemoteSession (Simulation)
AeroWorkflow
Setup
GeometricProperties
AirflowPhysics
SimulationConditions
ComponentGroups
Solution
Files
Solve
Results
Project
PostAnalysis
croot ()
Returns the current
RemoteSession
. If multiple Fluent connections exist, the current is related to the current selection in the Outline View.csim()
Returns the
croot().Case.App
of the current simulation.fcmd(cmd)
Execute a command in the current remote Fluent session. This can be used to execute Fluent style Text User Interface commands in the Fluent solver.
Example:
fcmd("/define/beta-feature-access yes ok")
feval(cmd)
Execute a command in the current remote Fluent session and return the result. This can be used to execute Fluent style Text User Interface commands in the Fluent solver.
The project framework is the top level container for all your simulation data, and
it is the first thing that must be accessed after opening Fluent Aero. A project must
first be created or loaded before any simulation is created, loaded or run. These
operations can be preformed from the Project ribbon. However, they can also be performed by using
the Project API commands, which can be typed directly into the python console, or
loaded from a python journal file. Project operations are defined in the
Project
object. To use a project API, type
Project
followed by the command name, [example:
Project.close()
]. If an operation fails, it will throw an
exception. Below is a list of some of the most common Project API commands that can
be used.
Project
new("filename")
Create a new project file in the specified path/filename, the path must be available. For example, Project.new("/tmp/DEMO") will create /tmp/DEMO.flprj and /tmp/DEMO.cffdb/
open("filename")
Open an existing project file.
close()
Close the current project.
erase("filename")
Erase the specified project, filename.flprj and filename.cffcb folder recursively.
isOpen()
Returns True if a project is currently opened.
getURL()
Returns the project file (.flprj) with full path.
getFolder()
Returns the full path of the project folder (cffdb).
openSimulation("name")
Load the specified simulation, by name, from the current project. Fluent is launched with the default.
newAeroWorkflow("file.cas")
Create a new simulation, importing the case file (.cas or .cas.h5, with path) to the project. This is equivalent to using the ribbon option , with default options.
saveCase()
Save the current settings to the case file (similar to
→ )
When using Fluent Aero in graphical mode (not in pure batch mode), the python
environment provides full access to the graphical capabilities of the Post-Analysis
module. The PostAnalysis
python module offers the related
services.
Note: Journaling capabilities for Post-Analysis are limited, and not all graphical operations will be recorded (camera configuration and movements, viewports, etc.)
Sample Usage
m = PostAnalysis.LoadCase(Filename=”naca.01.cas.h5”) d = PostAnalysis.LoadResult(Filename1=”out.0001.cas”,Filename2=”out.0002.dat”,FieldName=”Mach”) PostAnalysis.DisplaySettings.RestoreView(ViewName="bottom") PostAnalysis.Dataset[m].Case.Results.Graphics.Mesh["Mesh1"].Options.Edges = True PostAnalysis.Dataset[m].Case.Results.Graphics.Mesh["Mesh1"].Display() PostAnalysis.DisplaySettings.RestoreView(ViewName="front") PostAnalysis.Dataset[d].Case.Results.Graphics.Mesh["Contour1"].Display()
PostAnalysis
Init()
Initialize the post-processing framework (this will open the EnSight process and consume a post-processing license). Automatically invoked as required if
LoadCase
orLoadResult
are used.LoadCase(Filename="file.cas.h5”)
Loads the specified case or mesh file. The return value is the identifier of the newly loaded dataset and can be used as a key in the
PostAnalysis.Dataset[key]
index. .cas, and .cas.h5 can be loaded.LoadResult(Filename1="file.cas.h5”,Filename2=”file.dat.h5”)
LoadResult(Filename1="file.cas.h5”,Filename2=”file.dat.h5”,FieldName=”Fieldname”)
Loads the specified case and result. The return value is the identifier of the newly loaded dataset and can be used as a key in the
PostAnalysis.Dataset[key] index
. The .cas / .dat, .cas.h5 / .dat.h5, .cas.fsp /.dat.fsp must be loaded in matching pairs. If aFieldName
is provided, the specified fieldDataset
(Dictionary of loaded datasets -keys()
returns the list of dataset identifiers.Dataset[key].Case.Results
Object containing the dataset’s graphical elements and their settings. Use the
()
operator to query the content.DisplaySettings
Object containing the viewports and their display settings. Use the
()
operator to query the content, anddir()
to get the list of available commands.GlobalScene
Object containing the scenes and their display settings. Use the
()
operator to query the content.