The following two examples will illustrate how failing to specify a row and column position for each item in a table on dialog box can result in some interface elements being overwritten.
This example shows how a dialog box should look when all of
the elements are placed correctly in a table using the row
and col
arguments. The
four integer entry fields used in this example have both a row and
column specified in their create
statements.
This results in a clean and well structured dialog box. This dialog
box does not do anything when the button
is clicked because we have substituted Boolean values for the apply-cb
and update-cb
arguments,
which would normally be function calls. For more information on the apply-cb
and update-cb
functions,
see cx-create-panel.
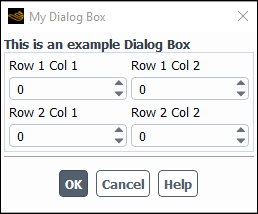
(define (apply-cb) #t) (define update-cb #f) (define table) (define int1) (define int2) (define int3) (define int4) (define my-dialog-box (cx-create-panel "My Dialog Box" apply-cb update-cb)) (set! table (cx-create-table my-dialog-box "This is an example Dialog Box")) (set! int1 (cx-create-integer-entry table "Row 1 Col 1" 'row 1 'col 1)) (set! int2 (cx-create-integer-entry table "Row 1 Col 2" 'row 1 'col 2)) (set! int3 (cx-create-integer-entry table "Row 2 Col 1" 'row 2 'col 1)) (set! int4 (cx-create-integer-entry table "Row 2 Col 2" 'row 2 'col 2)) (cx-show-panel my-dialog-box)
This example shows what happens when you decide not to use the
row and column arguments to position items in a table on your dialog
box. There are actually four integer entry fields on this dialog box,
but unfortunately the last integer entry field to be added to the
table doesn’t have a row and column position specified in its create
statement. As a result, the final integer entry
field will overwrite anything that is already in row 0 column 0. The
lesson to be learned here is to specify a position for every item
on you interface or else risk overwriting some elements with others.
This dialog box does not do anything when the button is clicked because we have substituted Boolean values for
the apply-cb
and update-cb
arguments, which would normally be function calls. For more information
on the apply-cb
and update-cb
functions, see cx-create-panel.
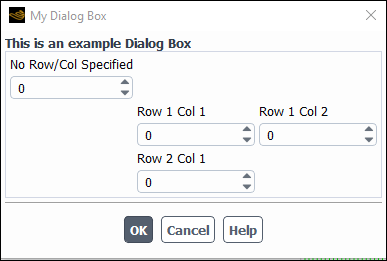
(define (apply-cb) #t) (define update-cb #f) (define table) (define int1) (define int2) (define int3) (define int4) (define my-dialog-box (cx-create-panel "My Dialog Box" apply-cb update-cb)) (set! table (cx-create-table my-dialog-box "This is an example Dialog Box")) (set! int1 (cx-create-integer-entry table "Row 1 Col 1" 'row 1 'col 1)) (set! int2 (cx-create-integer-entry table "Row 1 Col 2" 'row 1 'col 2)) (set! int3 (cx-create-integer-entry table "Row 2 Col 1" 'row 2 'col 1)) (set! int4 (cx-create-integer-entry table "No Row/Col Specified")) (cx-show-panel my-dialog-box)