This section contains the code for a dialog box that includes all of the features discussed in part two of this guide. This example can be used in conjunction with the UDF Example found later in this chapter in order to verify that the data is passed from the dialog box to the UDF using RP variables. This example contains comments in order to help readers understand the various parts of the example. All Scheme comment lines start with a semi-colon. This code automatically creates a menu called New Menu, with a submenu called New Submenu. The dialog box is automatically added as a menu item in New Submenu.
Note: In order for the List Options and Drop-Down List Options fields to populate, you must first read a mesh into Fluent because it uses information from the mesh as the list items.
This example uses a couple of Fluent macros that are not seen
previously in part two of this guide. The line (map thread-name(get-face-threads))
in the update-cb
function is used to populate
the list and drop-down list fields using face threads from your current
mesh when the dialog box is opened. As seen in chapter one, the Map statement applies a single function over
a list of data. This makes thread-name
the
function, which simply gets the name of the thread name as text from
the thread itself. The get-face-threads
part
of the statement is the list that the function is being applied to,
meaning that this statement returns a list of face threads from the
mesh on which the thread-name
function can
operate.
The other macro in this example that is not covered previously in part two of this guide is
the (%run-udf-apply)
macro. This macro is used because the
corresponding UDF Example uses a
DEFINE_EXECUTE_FROM_GUI
statement. This allows you to run your UDF
from the button of your GUI. The
(%run-udf-apply)
macro is used in the
apply-cb
function as a call to run the UDF. This macro requires one
integer parameter. This integer is used in the UDF to determine what the programmer asked it
to do. This is seen in the example below. The has a
different job then the button, so it passes the integer 1 instead of
2. When the UDF runs, it will test the value of that integer and decide what to do depending
on what the integer is. The DEFINE_EXECUTE_FROM_GUI
statement is the
only DEFINE
statement that uses a Scheme macro in the GUI code to
invoke the UDF. Other DEFINE
macros allow the UDF to be run at
various times during a simulation or, in the case of the
DEFINE_EXECUTE_ON_DEMAND
statement, whenever you want by
right-clicking User Defined Functions under the Parameters
& Customization branch of the tree and selecting . For more information on the various DEFINE
statements available for use in your UDF, see
DEFINE
Macros.
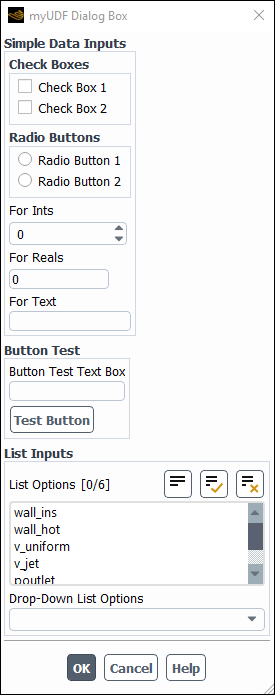
;RP Variable Declarations (make-new-rpvar 'myudf/real 0 'real) (make-new-rpvar 'myudf/int 0 'int) (make-new-rpvar 'myudf/checkbox1 #f 'boolean) (make-new-rpvar 'myudf/checkbox2 #f 'boolean) (make-new-rpvar 'myudf/radiobutton1 #f 'boolean) (make-new-rpvar 'myudf/radiobutton2 #f 'boolean) (make-new-rpvar 'myudf/string "" 'string) (make-new-rpvar 'myudf/buttonstr "" 'string) (make-new-rpvar 'myudf/list '() 'string-list) (make-new-rpvar 'myudf/droplist '() 'string-list) ;Dialog Box Definition (define gui-dialog-box ;Let Statement, Local Variable Declarations (let ((dialog-box #f) (table) (myudf/box1) (myudf/box2) (myudf/box3) (myudf/buttonbox1) (myudf/checkbox1) (myudf/checkbox2) (myudf/buttonbox2) (myudf/radiobutton1) (myudf/radiobutton2) (myudf/real) (myudf/int) (myudf/string) (myudf/buttonstr) (myudf/list) (myudf/droplist) ) ;Update-CB Function, Invoked When Dialog Box Is Opened (define (update-cb . args) (cx-set-toggle-button myudf/checkbox1 (rpgetvar 'myudf/checkbox1)) (cx-set-toggle-button myudf/checkbox2 (rpgetvar 'myudf/checkbox2)) (cx-set-toggle-button myudf/radiobutton1 (rpgetvar 'myudf/radiobutton1)) (cx-set-toggle-button myudf/radiobutton2 (rpgetvar 'myudf/radiobutton2)) (cx-set-integer-entry myudf/int (rpgetvar 'myudf/int)) (cx-set-real-entry myudf/real (rpgetvar 'myudf/real)) (cx-set-text-entry myudf/string (rpgetvar 'myudf/string)) (cx-set-text-entry myudf/buttonstr (rpgetvar 'myudf/buttonstr)) (cx-set-list-items myudf/list (map thread-name(get-face-threads))) (cx-set-list-selections myudf/list (rpgetvar 'myudf/list)) (cx-set-list-items myudf/droplist (map thread-name(get-face-threads))) (cx-set-list-selections myudf/droplist (rpgetvar 'myudf/droplist)) ) ;Apply-CB Function, Invoked When "OK" Button Is Clicked (define (apply-cb . args) (rpsetvar 'myudf/checkbox1 (cx-show-toggle-button myudf/checkbox1)) (rpsetvar 'myudf/checkbox2 (cx-show-toggle-button myudf/checkbox2)) (rpsetvar 'myudf/radiobutton1 (cx-show-toggle-button myudf/radiobutton1)) (rpsetvar 'myudf/radiobutton2 (cx-show-toggle-button myudf/radiobutton2)) (rpsetvar 'myudf/int (cx-show-integer-entry myudf/int)) (rpsetvar 'myudf/real (cx-show-real-entry myudf/real)) (rpsetvar 'myudf/string (cx-show-text-entry myudf/string)) (rpsetvar 'myudf/buttonstr (cx-show-text-entry myudf/buttonstr)) (rpsetvar 'myudf/list (cx-show-list-selections myudf/list)) (rpsetvar 'myudf/droplist (cx-show-list-selections myudf/droplist)) (%run-udf-apply 2) ) ;Button-CB Function, Invoked When "Test Button" Is Clicked (define (button-cb . args) (rpsetvar 'myudf/buttonstr (cx-show-text-entry myudf/buttonstr)) (%run-udf-apply 1) ) ;Args Function, Used For Interface Setup, Required For Apply-CB, Update-CB, and Button-CB Sections (lambda args (if (not dialog-box) (let () (set! dialog-box (cx-create-panel "myUDF Dialog Box" apply-cb update-cb)) (set! table (cx-create-table dialog-box "" 'border #f 'below 0 'right-of 0)) (set! myudf/box1 (cx-create-table table "Simple Data Inputs" 'row 0)) (set! myudf/box2 (cx-create-table table "Button Test" 'row 1)) (set! myudf/box3 (cx-create-table table "List Inputs" 'row 2)) (set! myudf/buttonbox1 (cx-create-button-box myudf/box1 "Check Boxes" 'radio-mode #f 'row 0)) (set! myudf/checkbox1 (cx-create-toggle-button myudf/buttonbox1 "Check Box 1")) (set! myudf/checkbox2 (cx-create-toggle-button myudf/buttonbox1 "Check Box 2")) (set! myudf/buttonbox2 (cx-create-button-box myudf/box1 "Radio Buttons" 'radio-mode #t 'row 1)) (set! myudf/radiobutton1 (cx-create-toggle-button myudf/buttonbox2 "Radio Button 1")) (set! myudf/radiobutton2 (cx-create-toggle-button myudf/buttonbox2 "Radio Button 2")) (set! myudf/int (cx-create-integer-entry myudf/box1 "For Ints" 'row 2)) (set! myudf/real (cx-create-real-entry myudf/box1 "For Reals" 'row 3)) (set! myudf/string (cx-create-text-entry myudf/box1 "For Text" 'row 4)) (set! myudf/buttonstr (cx-create-text-entry myudf/box2 "Button Test Text Box" 'row 0)) (cx-create-button myudf/box2 "Test Button" 'activate-callback button-cb 'row 1) (set! myudf/list (cx-create-list myudf/box3 "List Options" 'visible-lines 5 'multiple-selections #t 'row 0)) (cx-set-list-items myudf/list (map thread-name (sort-threads-by-name(get-face-threads)))) (set! myudf/droplist (cx-create-drop-down-list myudf/box3 "Drop-Down List Options" 'multiple-selections #f 'row 1)) (cx-set-list-items myudf/droplist (map thread-name (sort-threads-by-name(get-face-threads)))) ) ;End Of Let Statement ) ;End Of If Statement ;Call To Open Dialog Box (cx-show-panel dialog-box) ) ;End Of Args Function ) ;End Of Let Statement ) ;End Of GUI-Dialog-Box Definition (cx-add-menu "New Menu" #f) (cx-add-hitem "New Menu" "New Submenu" #f #f #t #f) ;Menu Item Added To Above Created "New Menu->New Submenu" Submenu In Fluent (cx-add-item "New Submenu" "MyUDF Dialog Box" #\U #f #t gui-dialog-box)