To control state handling, you can access state values from the
Ansys.ACT.Interfaces.Common.State
enumeration.
The following table lists state-handling APIs.
Class | Member | Description |
---|---|---|
Ansys.ACT.Interfaces.Common.State | UpToDate | The task is in an up-to-date state. |
RefreshRequired | The task requires a refresh to continue upstream changes. | |
OutOfDate | The task is in an out-of-date state. This state is valid only in Mechanical. | |
UpdateRequired | The task must be updated. | |
Modified | The task has been modified. This state is valid only in Mechanical. | |
Unfulfilled | Task requirements are unfulfilled. | |
Disabled | The task is disabled. | |
Error | The task is in error. | |
EditRequired | Data entered for the task is invalid or incomplete and must be modified. | |
Interrupted | Processing of the task is halted. | |
UpstreamChangesPending | Updating of the task cannot be completed until previous tasks are updated. | |
Unknown | The state of the task could not be determined. |
When defining the state callback on tasks, you can return the framework-defined
ComponentState
instance. However, returning this instance is
not recommended unless you must maintain backwards compatibility. Instead, return a
two-entry list, where the first entry is an
Ansys.ACT.Interfaces.Common.State
enumeration value and the
second entry is a quick-help string.
import clr clr.AddReference('Ansys.ACT.Interfaces') import Ansys.ACT.Interfaces def status(task): if task.Properties['Inputs'].Properties['Input'].Value == 1: return [ Ansys.ACT.Interfaces.Common.State.Unfulfilled , 'cannot enter the value of 1' ] else: return None #rely on the default framework-calculated state
When executed, this code sample yields the following result:
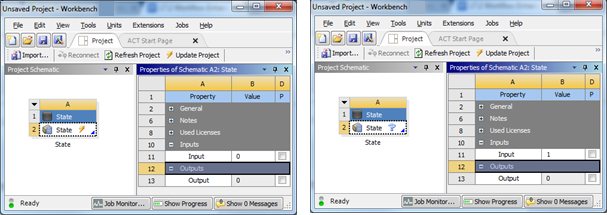
In addition, ACT provides a default state handler whenever the task does not provide a
callback <onstatus>
. When any task property is invalid, ACT
instructs the framework to use an unfulfilled state, as shown in the following examples
of an XML file and IronPython script.
- XML File:
<extension version="1" name="StateValidity"> <guid shortid="StateValidity"> 69d0155b-e138-4841-a13a-de12238c83f2 </guid> <script src="main.py" /> <interface context="Project"> <images>images</images> </interface> <workflow name="MyWorkflow" context="Project" version="1"> <tasks> <task name="StateValidity" caption="State Validity" icon="Generic_cell" version="1"> <callbacks> <onupdate>update</onupdate> <onreset>reset</onreset> </callbacks> <property name="Valid" caption="Property Check" control="integer" default="0" readonly="false" needupdate="true" visible="true" persistent="true" isparameter="true"> <callbacks> <isvalid>valid</isvalid> </callbacks> </property> <property name="Input" caption="Input" control="float" default="0.0" readonly="false" needupdate="true" visible="true" persistent="true" isparameter="true" /> <property name="Output" caption="Output" control="float" default="0.0" readonly="true" visible="true" persistent="true" isparameter="true" /> <inputs> <input/> </inputs> <outputs/> </task> </tasks> <taskgroups> <taskgroup name="StateValidity" caption="State Validity" icon="Generic" category="" abbreviation="State" version="1"> <includeTask name="StateValidity" caption="State Validity"/> </taskgroup> </taskgroups> </workflow> </extension>
- IronPython Script:
import clr clr.AddReference('Ansys.ACT.Interfaces') import Ansys.ACT.Interfaces def update(task): activeDir = task.ActiveDirectory extensionDir = task.Extension.InstallDir exeName = "ExampleAddinExternalSolver.exe" solverPath = System.IO.Path.Combine(extensionDir, exeName) inputValue = task.Properties["Inputs"].Properties["Input"].Value inputFileName = "input.txt" outputFileName = "output.txt" dpInputFile = System.IO.Path.Combine(activeDir, inputFileName) dpOutputFile = System.IO.Path.Combine(activeDir, outputFileName) #write input file f = open(dpInputFile, "w") f.write('input='+inputValue.ToString(System.Globalization.NumberFormatInfo.InvariantInfo)) f.close() exitCode = ExtAPI.ProcessUtils.Start(solverPath, dpInputFile, dpOutputFile) if exitCode != 0: raise Exception ('External solver failed!') #read output file outputValue = None f = open(dpOutputFile, "r") currLine = f.readline() while currLine != "": valuePair = currLine.split('=') outputValue = System.Double.Parse(valuePair[1],System.Globalization.NumberFormatInfo.InvariantInfo) currLine = f.readline() f.close() if outputValue == None: raise Exception("Error in update - no output value detected!") else: task.Properties["Output"].Value = outputValue def reset(task): task.Properties["Input"].Value = 0 task.Properties["Output"].Value = 0 def valid(entity, property): if property.Value == 1: return False else: return True
- Result: