ACT fully wraps the framework’s reporting API. To generalize APIs across all Ansys products, interfaces have been defined. They can potentially be used to adopt reporting for other products outside of Workbench.
The following table lists project reporting APIs.
Class | Member | Description |
---|---|---|
IReport | AddChild(item) | Adds a child report item to the report. |
AddChild(item, index) | Adds a child report item to the report. | |
RemoveChild(index) | Removes a child report item from the report. | |
GetChild(index) | Obtains a child report item from the report. | |
ChildCount | The number of children inside the report. | |
FilePath | The report file path. | |
ImageDirectoryPath | The image directory path for report images. | |
ProjectReport | AddReportImage(name) | Adds an image item to the report. |
InsertReportImage(name, index) | Inserts an image item into the report. | |
AddReportLink(name) | Adds a link item to the report. | |
InsertReportLink(name, index) | Inserts a link item into the report. | |
AddReportSection(name) | Adds a section item to the report. | |
InsertReportSection(name, index) | Inserts a section item into the report. | |
AddReportText(name) | Adds a text item to the report. | |
InsertReportText(name, index) | Inserts a text item into the report. | |
AddReportTable(name) | Adds a table item to the report. | |
AddReportTable(name, numberOfRows,
numberOfColumns) | Adds a table item to the report, with the specified number of rows and columns. | |
InsertReportTable(name, index) | Inserts a table item into the report. | |
IReportItem | Name | The name of the report item. |
ProjectReportImage | ProjectReportImage(name) | Constructor. |
ProjectReportImage(name,
sourceLocation) | Constructor. | |
SourceLocation | The image location (file path). | |
ProjectReportLink |
ProjectReportLink(name) | Constructor. |
ProjectReportLink(name,
resourceLocation) | Constructor. | |
ResourceLocation | The linked path. | |
ProjectReportSection | ProjectReportSection() | Constructor. |
ProjectReportSection(name) | Constructor. | |
AddChild(item) | Adds a child report item to the report section. | |
AddChild(item, index) | Adds a child report item to the report section at the specified index. | |
RemoveChild(index) | Removes a child report item from the section at the given index. | |
GetChild(index) | Retrieves a child report item from the section at the given index. | |
ChildCount | The number of children inside the report section. | |
AddReportImage(name) | Adds an image item to the report. | |
InsertReportImage(name, index) | Inserts an image item into the report section. | |
AddReportLink(name) | Adds a link item to the report section. | |
InsertReportLink(name, index) | Inserts a link item into the report section. | |
AddReportSection(name) | Adds a section item to the report section. | |
InsertReportSection(name, index) | Inserts a section item into the report section. | |
AddReportText(name) | Adds a text item to the report section. | |
InsertReportText(name, index) | Inserts a text item into the report section. | |
AddReportTable(name) | Adds a table item to the report section. | |
AddReportTable(name, numberOfRows,
numberOfColumns) | Adds a table item to the report section, with the specified number of rows and columns. | |
InsertReportTable(name, index) | Inserts a table item into the report section. | |
ProjectReportTable | ProjectReportTable(name) | Constructor. |
ProjectReportTable(name, numRows,
numColumns) | Constructor. | |
RowCount | The number of rows in the table. | |
ColumnCount | The number of columns in the table. | |
GetColumnName(index) | Retrieves the name for a column at the specified index. | |
SetColumnName(index) | Sets the name for a column at the specified index. | |
AddColumn(columnName) | Adds an empty column to the table using the supplied column name. | |
AddColumn(columnName, index) | Adds an empty column to the table at the specified index using the supplied column name. | |
AddColumn(columnName, index,
columnValues) | Adds a column to the table at the specified index using the supplied column name and populated with the supplied values. | |
RemoveColumn(index) | Removes a column at the specified index. | |
AddRow(rowValues) | Adds a new row of values to the table. | |
AddRow(rowValues, index) | Adds a new row of values to the table at the specified index. | |
RemoveRow(index) | Removes a row from the table at the specified index. | |
GetValue(rowIndex, columnIndex) | Retrieves a value from the table at the specified row-and-column coordinates. | |
SetValue(rowIndex, columnIndex, value) | Sets a report table value in the table at the supplied row-and-column coordinates. | |
SetValue(rowIndex, columnIndex,
content) | Sets a string value in the table at the supplied row-and-column coordinates. | |
SetRowBackgroundColor(index, color) | Sets the background color for the row at the specified index. | |
GetRowBackgroundColor(index) | Retrieves the current row background color for the row at the supplied index. | |
SetRowForegroundColor(index, color) | Sets the foreground color for the row at the specified index. | |
GetRowForegroundColor(index) | Retrieves the current row foreground color for the row at the supplied index. | |
ShowHeaders | Indicates whether or not the column headers should be displayed. | |
IsCollapsible | Indicates whether or not the table is collapsible. | |
ProjectReportTableValue | ProjectReportTableValue(content) | Constructor. |
ProjectReportTableValue(content, iconSourceLocation,
iconAlternateText) | Constructor. | |
Content | The table value string. | |
IconSourceLocation | The cell icon source path. | |
IconAlternateText | The cell icon alternate text to use when the icon cannot be located. | |
ProjectReportText | ProjectReportText(text) | Constructor. |
ProjectReportText(name, text) | Constructor. | |
Text | Gets or sets the report item’s text. |
Consider the following code sample:
import clr clr.AddReference("Ansys.ACT.WorkBench") import Ansys.ACT.WorkBench def report(task, report): section = report.AddReportSection("My Custom ACT Task Report Content") text = section.AddReportText("TestText") text.Text = "Sample text from the data squares component" link = section.AddReportLink("TestLink") link.ResourceLocation = "http://www.ansys.com" extDir = ExtAPI.ExtensionManager.CurrentExtension.InstallDir imgDir = System.IO.Path.Combine(extDir, "images") imgName = "logo-ansys.jpg" imgPath = System.IO.Path.Combine(imgDir, imgName) reportImgDir = report.ImageDirectoryPath destImgPath = System.IO.File.Copy(imgPath, System.IO.Path.Combine(reportImgDir, imgName)) img = section.AddReportImage("TestImage") img.SourceLocation = imgName rowCount = 5 colCount = 3 table = section.AddReportTable("TestTable", rowCount, colCount) for i in range(0, rowCount): evenRow = (i % 2 == 0) rowVals = [] for j in range(0, colCount): table.SetValue(i, j, str(j + i*colCount)) if evenRow: table.SetRowBackgroundColor(i, "Red") table.SetRowForegroundColor(i, "Blue") else: table.SetRowBackgroundColor(i, "Blue") table.SetRowForegroundColor(i, "Red") table.SetColumnName(0, "Col 1") table.SetColumnName(1, "Col 2") table.SetColumnName(2, "Col 3")
When executed, this code sample generates the following report:
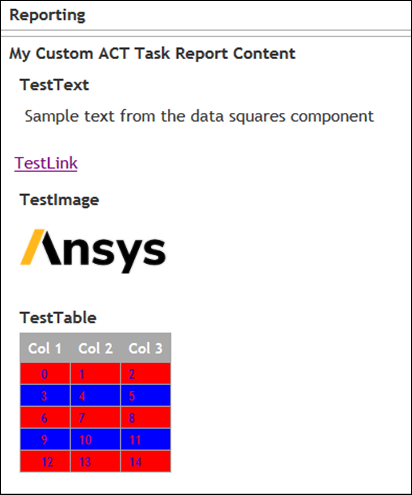