Using the InputHelper
The InputHelper enables you to pause the execution of the script to get various inputs from interactive tools, before continuing to run the script. You can set up custom workflows using the InputHelper functions, including creating widgets and tool guides for user input.
You can create tool guides and specify selections which can also be filtered based on type. For example, you can choose to filter faces, edges, or bodies. You can also specify if the selections are mandatory or optional.
Script variables can be added to the Options panel as buttons, check boxes, drop-downs, or groups of such controls. You can also add tool tips for the controls created.
Example - Creating Tool Guides for Selection
This example shows how you can select a body to be moved and select edges to be rounded.
s1 = InputHelper.CreateSelection('Body (Required)', SelectionFilter.Body,True) s2 = InputHelper.CreateSelection('Edge (2 Required)', SelectionFilter.Edge, 2, [True]) s3 = InputHelper.CreateSelection('Edge or face (optional)', SelectionFilter.Edge|SelectionFilter.Face) InputHelper.PauseAndGetInput('Select body to move. Select edges for rounding.', s1, s2, s3) # Translate Along X Handle selection = s1.Value direction = Direction.DirX options = MoveOptions() result = Move.Translate(selection, direction, MM(30), options) # EndBlock # Create 1 Round selection = s2.Value options = ConstantRoundOptions() result = ConstantRound.Execute(selection, MM(1), options) # EndBlock
The script pauses to start the interactive tool, where you can select a body to be moved and two edges to be rounded.
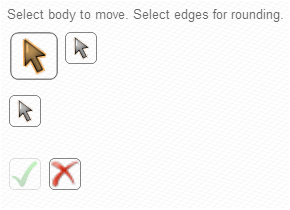
When you finish selecting the entities, you can click Complete or Abort
and resume the
script.
Example - Creating Input Options
This example shows how you can specify variables as options to be displayed in the options panel.
def Test(item): if x==1 : return True else: return False # User defined function validation method for a selection function = InputHelper.CreateSelection(Test, 'test') #Examples of the options for the InputHelper box = InputHelper.CreateButton(CreateBox, 'Create Box', 'Creates a box of unit dimensions') prompt = InputHelper.CreateCheckBox(False, 'Quick Actions', 'Enable Quick Actions') color = InputHelper.CreateColorDropDown(Color.Aqua, 'Color', 'Set color ') list = InputHelper.CreateComboBox("Options", "Choose One", "a", "b", "c") # Options Group Creation name = InputHelper.CreateTextBox('abc', 'Name', 'Enter Name') angle = InputHelper.CreateTextBox(1, 'Angle', 'Enter Angle', ValueType.Angle) length = InputHelper.CreateTextBox(0.001, 'Length', 'Enter Length', ValueType.Length) settings = InputHelper.CreateOptionsGroup('Settings', name,angle,length) InputHelper.PauseAndGetInput('Select object', box, prompt,color,list,settings,selection)
The script pauses to start the interactive tool, where you can select an object (a face or edge in this example) and specify variables in the Options panel.
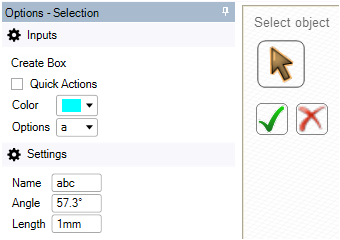
When you finish specifying the variables, you can click Complete or Abort
and resume the
script.