Changing Settings
'IAS_': Interface to Analysis Settings.
ZOSAPI is built on the concept of 'Interfaces'. (We leave finding a deeper understanding of Interfaces as an exercise for the Reader.)
As an experienced OpticStudio User, you know that each Analysis has its own group of 'Settings'. In ZOSAPI, each Analysis's Settings are defined in an Interface and, in order for the compiler to understand what you're doing, you have to tell the compiler which Interface you are using. OpticStudio has also placed each of the Analyses which are normally 'grouped' together into their own 'namespaces'. For example, for the FftMtf Analysis, you need to add another 'using' statement at the top of your file:
using ZOSAPI.Analysis.Mtf;
The line of code that provides access to the settings of the FftMtf Analysis is:
IAS_FftMtf FftMtf_Settings = FftMtf.GetSettings() as IAS_FftMtf;
You 'read' this as:
Using your Analysis (variable FftMtf), assign the Settings to variable FftMtf_Settings and use the 'Interface to Analysis Settings for the FftMtf Analysis' to access the data therein.
NOTE: While it is possible to use the C# language 'type' 'var' it is less unwise to use the actual type name to catch, at compile time, any typing errors you might make.
Settings with 'built-in' types
Values of settings with built-in types (bool, int, double, etc...) are used as simply as one would expect:
FftMtf_Settings.ShowDiffractionLimit = true;
FftMtf_Settings.UsePolarization = false;
FftMtf_Settings.MaximumFrequency = 42.0;
bool fftIsUsingDashes = FftMtf_Settings.UseDashes;
Settings That Have Discrete Values
These are settings that, in the OpticStudio UI, are set using a choice from a list of choices (the OpticStudio UI displays them in a drop down box). The 'list of choices' are defined as members of an 'enum' and the setting is defined using that enum as its type. Thus:
MtfTypes currentType = FftMtf_Settings.Type;
FftMtf_Settings.Type = MtfTypes.SquareWave;
FftMtf_Settings.SampleSize = SampleSizes._128x128;
An example of the 'list of choices':
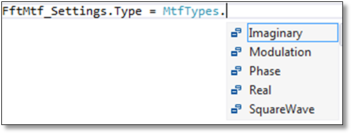
Fields
Fields use functions to get and set their values. There is also other functionality available for Fields. The 'set' functions return an 'IMessage' which contains any errors that happen when you try to set a Field to an illegal value.
int nF_FftMtf = FftMtf_Settings.Field.GetFieldNumber(); IMessage iM_SetFieldN = FftMtf_Settings.Field.SetFieldNumber(nF + 11); IMessage iM_UseAllFields = FftMtf_Settings.Field.UseAllFields();
Wavelengths
Wavelengths use functions to get and set their values. There is also other functionality available for Wavelengths. The 'set' functions return an 'IMessage' which contains any errors that happen when you try to set a Wavelength to an illegal value.
int numW = FftMtf_Settings.Wavelength.GetWavelengthNumber(); IMessage iM_WaveN = FftMtf_Settings.Wavelength.SetWavelengthNumber(11); IMessage iM_UseAllWaves = FftMtf_Settings.Wavelength.UseAllWavelengths();
Surfaces
Surfaces use functions to get and set their values. There is also other functionality available for Surfaces. The 'set' functions return an 'IMessage' which contains any errors that happen when you try to set a Surface to an illegal value.
int nS = FftMtf_Settings.Surface.GetSurfaceNumber(); IMessage iM_SetSurfN = FftMtf_Settings.Surface.SetSurfaceNumber(22); IMessage iM_UseImageSurf = FftMtf_Settings.Surface.UseImageSurface(); IMessage iM_UseObjSurf = FftMtf_Settings.Surface.UseObjectiveSurface();
Next: